23 Advanced but Useful Git Commands and Techniques
A comprehensive guide to advanced Git techniques and commands, enhancing your version control skills.
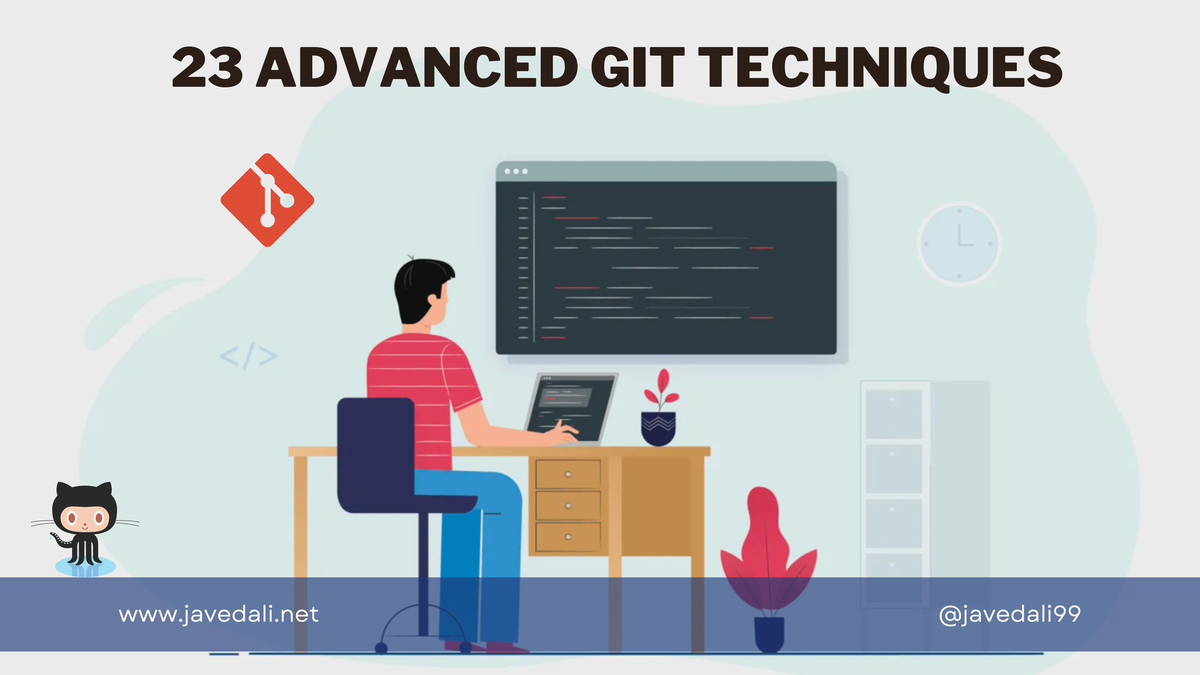
In the ever-evolving world of software development, mastering the art of version control is a non-negotiable skill. Git, a distributed version control system, is a fundamental tool that every developer must get comfortable with. It allows multiple people to work on the same project without stepping on each other’s toes, and it keeps a history of all changes, making it easier to track and undo modifications. This post will delve into 23 advanced Git techniques and commands that can help you become more efficient and productive. These techniques will take your Git skills to the next level.
1. Git Add and Commit in One Step
Instead of using git add
followed by git commit
to save a snapshot of your code, you can combine these two steps into one. The -am
flag with git commit
automatically adds all the files in the current working directory and commits them. This command is a time-saver and keeps your Git history clean.
git commit -am "Your commit message"
2. Creating Git Aliases
Git config allows you to create aliases, which can be used to shorten existing commands or create new custom commands. For instance, you could create an alias ac
that runs the add and commit command with just two letters.
git config --global alias.ac 'commit -am'
3. Correcting Mistakes with Amend
If you made a typo in your last commit message or forgot to include some files, you can use the --amend
flag to update the latest commit. If you want to keep the same commit message, add the --no-edit
flag.
git commit --amend -m "New commit message"
git commit --amend --no-edit
4. Reverting Commits
If you pushed some code to a remote repository and then realized it was a mistake, you can use the git revert
command to go back to the state of the previous commit. This doesn’t remove the original commit from the history; instead, it creates a new commit that undoes the changes made in the original commit.
git revert <commit_id>
5. Using GitHub’s Web-Based VS Code
If you need to work on a repo but don’t have access to your local machine, you can use GitHub’s web-based version of VS Code. Simply go to the repo on GitHub and hit the period key on your keyboard. This opens a browser-based version of VS Code where you can make edits and submit pull requests.
6. Stashing Changes
The git stash
command allows you to save changes that you’re not ready to commit yet. These changes are removed from your current working directory and can be reapplied later using git stash pop
.
git stash
git stash pop
7. Renaming the Master Branch
Post-2020, the term “master” is no longer the preferred nomenclature for the default branch in Git. Instead, use terms like “main”, “mega”, or “mucho”. You can rename the branch using git branch -m
.
git branch -m old_name new_name
8. Reading Git Log
The git log
command shows a history of commits. To make the output more readable, add the options --graph
, --oneline
, and --decorate
.
git log --graph --oneline --decorate
9. Using Git Bisect
The git bisect
command can help you pinpoint the exact commit that introduced a bug, saving you time and effort in the debugging process. It performs a binary search between a known good commit and a bad commit, helping you identify exactly which code needs to be fixed. You first need to know a good commit (one where the issue doesn’t exist) and a bad commit (one where the issue does exist). Then, you can run git bisect start
and git bisect bad
to mark the current commit as bad. Then, you can check out an older commit and mark it as good with git bisect good
. Git Bisect will then automatically narrow down the range of commits to check, asking you to test each one until you find the commit that introduced the issue.
git bisect start
git bisect good <good_commit_id>
git bisect bad <bad_commit_id>
10. Squashing Commits
If you have multiple commits on a feature branch that you want to merge into the main branch, you can squash them into a single commit using git rebase -i
.
git rebase -i HEAD~<number_of_commits>
11. Using Git Hooks
Git hooks allow you to run code before or after certain Git events. For example, you could configure a hook to validate or lint your code before each commit, improving your overall code quality.
# .git/hooks/pre-commit
npm run lint
12. Resetting to a Remote State
If you want to discard all local changes and reset your local repository to match the remote repository, you can use git fetch
followed by git reset --hard
.
git fetch origin
git reset --hard origin/main
13. Checking Out the Previous Branch
If you recently switched out of a branch and forgot its name, you can use git checkout -
to go directly back to the previous branch you were working on.
git checkout -
14. Git Archive
The git archive
command allows you to create a compressed archive of the files in your Git repository at a specific commit. This is useful when you want to share your code with someone who doesn’t use Git or when you want to create a ZIP or TAR archive of your code without the Git history.
git archive --format=<format> <commit> -o <filename>
15. Git Clean
The git clean
command allows you to remove untracked files from your working directory. These could be temporary files, build artifacts, or other files that you don’t need. You can also use options like -n
to preview which files will be deleted before actually deleting them, and -d
to delete untracked directories as well as files.
git clean
16. Git Grep
The git grep
command lets you search for specific text or patterns within your repository. This can be helpful when you need to find all instances of a particular function, variable, or string across your project.
git grep <search-term>
17. Git Submodule
With git submodule
, you can include a specific version of another project within your own project, ensuring that both projects' versions are maintained independently. This is a great way to manage dependencies and keep your project organized.
git submodule add [repository URL] [destination folder]
18. Git Worktree
The git worktree
command allows you to have multiple working trees or checkouts of the same repository on your local machine. This means you can work on different branches or even different repositories at the same time without having to switch back and forth.
git worktree add [path] [branch/commit]
19. Git Blame
The git blame
command allows you to see who made each change to a file, making it easy to track down the original author of a particular line of code. This can be especially useful when working with large codebases or collaborating with other developers.
git blame <file>
20. Git Cherry Pick
The git cherry-pick
command is useful when applying changes from one branch to another without merging the entire branch. It can be helpful in situations where you need to apply a hotfix to a production branch or when you want to move specific changes between branches.
git cherry-pick <commit-hash>
21. Git Diff
The git diff
command allows you to compare the changes between two commits or two branches. It shows the differences between the files, highlighting the lines that were added or removed.
git diff <commit1> <commit2>
22. Git Rebase
The git rebase
command allows you to move your branch to a new base commit. It helps you apply changes made to one branch to another branch, making it easier to keep your branch up-to-date with the latest changes from the main branch.
git rebase <base>
23. Git Reflog
The git reflog
command shows a list of all the actions you’ve taken in your Git repository, including commits, merges, and resets. It’s useful when you’ve made a mistake and need to undo a previous action or find a lost commit.
git reflog
Mastering these advanced Git techniques can significantly enhance your productivity as a developer. They can help you navigate your codebase with ease, collaborate more effectively with your team, and save you precious time. Happy coding! 💻🎉